Introduction
JavaScript is one of the most widely used programming languages in web development, powering everything from interactive user interfaces to backend logic. However, writing clean and efficient JavaScript code is crucial for maintainability, scalability, and performance. Poorly written code can lead to debugging nightmares, slow applications, and frustrated developers.
This guide will explore best practices, proper code structuring, and common mistakes to avoid when writing JavaScript. Whether you’re a beginner or an experienced developer, these tips will help you improve the readability, efficiency, and maintainability of your JavaScript code.
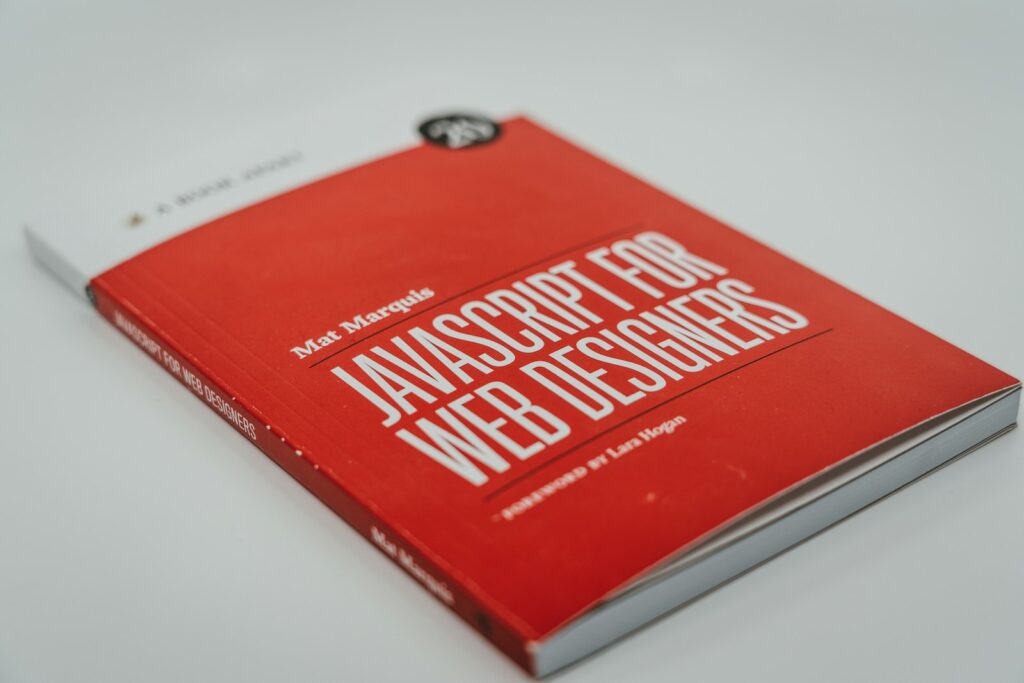
1. Follow a Consistent Code Style
1.1 Use Proper Naming Conventions
Good variable and function names make your code easier to read and understand. Follow these conventions:
- Variables: Use camelCase (e.g.,
userAge
,totalPrice
). - Functions: Use verbs to describe what they do (e.g.,
getUserData()
,calculateTotal()
). - Constants: Use UPPER_CASE_SNAKE_CASE (e.g.,
MAX_USERS
,API_BASE_URL
).
Bad Example:
javascriptCopyEditlet x = 10;
function a() { return x * x; }
Good Example:
javascriptCopyEditlet squareSide = 10;
function calculateArea() { return squareSide * squareSide; }
1.2 Use Consistent Formatting
Use consistent indentation, spacing, and line breaks to improve readability. A prettifier like Prettier can automate this process.
Bad Example:
javascriptCopyEditfunction sum(a,b){return a+b;}
Good Example:
javascriptCopyEditfunction sum(a, b) {
return a + b;
}
2. Write Modular and Reusable Code
2.1 Use Functions to Avoid Repetition
Avoid writing duplicate code by breaking logic into reusable functions.
Bad Example:
javascriptCopyEditlet area1 = 10 * 10;
let area2 = 15 * 15;
Good Example:
javascriptCopyEditfunction calculateArea(side) {
return side * side;
}
let area1 = calculateArea(10);
let area2 = calculateArea(15);
2.2 Use Modules for Code Organization
Instead of writing everything in one file, break your code into separate modules for better maintainability.
Example:
math.js
javascriptCopyEditexport function add(a, b) {
return a + b;
}
export function multiply(a, b) {
return a * b;
}
main.js
javascriptCopyEditimport { add, multiply } from './math.js';
console.log(add(5, 3)); // Output: 8
console.log(multiply(5, 3)); // Output: 15
3. Optimize Loops and Iterations
3.1 Use forEach()
Instead of for
Loops
For cleaner and more readable code, use higher-order functions like forEach()
, map()
, and reduce()
instead of traditional for
loops.
Bad Example:
javascriptCopyEditlet numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i] * 2);
}
Good Example:
javascriptCopyEditnumbers.forEach(num => console.log(num * 2));
3.2 Use map()
Instead of Manually Transforming Arrays
Bad Example:
javascriptCopyEditlet doubled = [];
for (let i = 0; i < numbers.length; i++) {
doubled.push(numbers[i] * 2);
}
Good Example:
javascriptCopyEditlet doubled = numbers.map(num => num * 2);
4. Handle Errors Properly
4.1 Use try...catch
for Error Handling
Bad Example:
javascriptCopyEditlet data = JSON.parse(userInput); // This will crash if `userInput` is invalid JSON
Good Example:
javascriptCopyEdittry {
let data = JSON.parse(userInput);
} catch (error) {
console.error("Invalid JSON format:", error.message);
}
4.2 Use Default Values and Optional Chaining
Instead of manually checking for undefined
, use optional chaining (?.
) and default values (||
).
Example:
javascriptCopyEditlet user = {};
console.log(user.profile?.name || "Guest"); // Output: "Guest"
5. Improve Performance
5.1 Avoid Unnecessary DOM Manipulations
Repeatedly modifying the DOM slows down performance. Use DocumentFragment or batch updates.
Bad Example:
javascriptCopyEditlet list = document.getElementById("list");
["A", "B", "C"].forEach(item => {
let li = document.createElement("li");
li.textContent = item;
list.appendChild(li);
});
Good Example:
javascriptCopyEditlet list = document.getElementById("list");
let fragment = document.createDocumentFragment();
["A", "B", "C"].forEach(item => {
let li = document.createElement("li");
li.textContent = item;
fragment.appendChild(li);
});
list.appendChild(fragment);
5.2 Use debounce()
for Optimizing Events
If handling frequent events like scrolling or keypress, use debouncing to improve efficiency.
Example:
javascriptCopyEditfunction debounce(func, delay) {
let timer;
return function(...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
window.addEventListener("resize", debounce(() => console.log("Resized!"), 300));
6. Avoid Common Mistakes
6.1 Avoid Global Variables
Global variables can cause conflicts and make debugging difficult. Always use let
, const
, or modules instead of polluting the global scope.
6.2 Use ===
Instead of ==
Always use strict equality (===
) to avoid unexpected type conversions.
Bad Example:
javascriptCopyEditconsole.log(5 == "5"); // true (bad practice)
Good Example:
javascriptCopyEditconsole.log(5 === "5"); // false (correct)
7. Use Modern JavaScript Features
7.1 Use Destructuring
Instead of manually extracting values, use destructuring for cleaner code.
Example:
javascriptCopyEditconst user = { name: "John", age: 30 };
const { name, age } = user;
console.log(name, age);
7.2 Use Template Literals
Example:
javascriptCopyEditlet name = "Alice";
console.log(`Hello, ${name}!`);
Conclusion
Writing clean and efficient JavaScript code is not just about making it work—it’s about making it maintainable, scalable, and readable. By following best practices such as consistent formatting, modular coding, efficient loops, error handling, and performance optimizations, you can significantly improve the quality of your JavaScript code.
By applying these techniques, you’ll not only write better code but also make collaboration and debugging much easier. Happy coding!
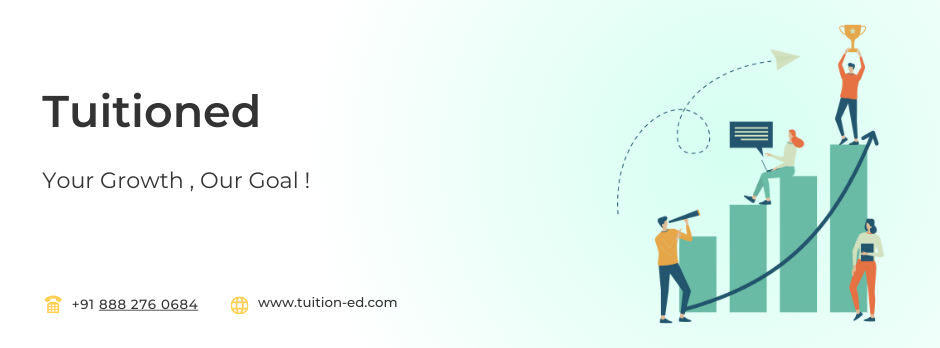